Getting Started With Jakarta EE - A Sample Application
Jakarta EE 8 has officially been released last Tuesday. It's the beginning of what it's been promised as "the Future of Cloud Native Java". Let's look at a sample application in Jakarta EE, using Wildfly, Gradle and Docker.
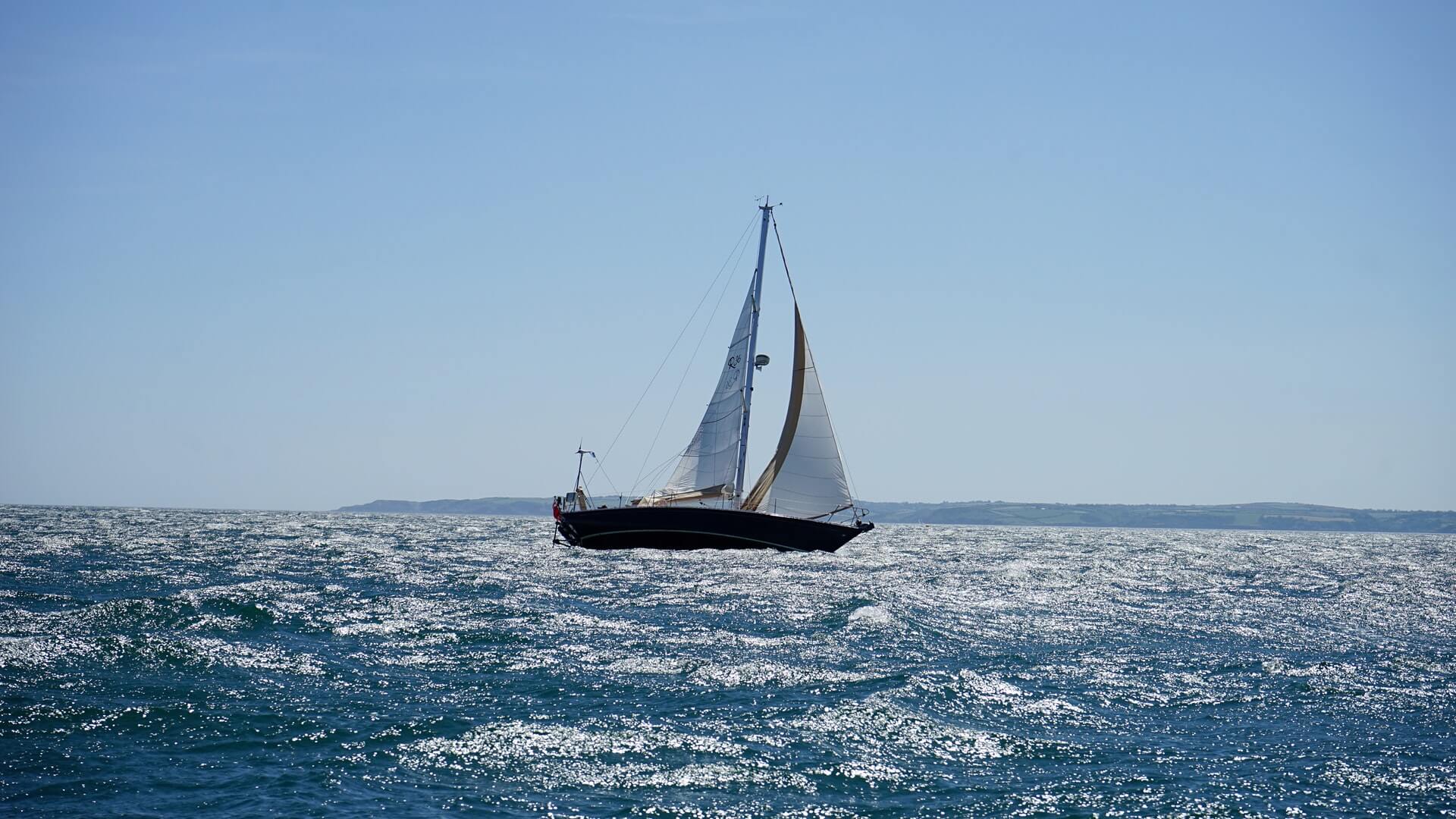
Jakarta EE 8 has officially been released last Tuesday. It's the beginning of what it's been promised as "the Future of Cloud Native Java". The release doesn't bring any new feature, but it's fully compatible with Java EE 8, as Mike Millinkovich explains in his article:
The Jakarta EE 8 specifications are fully compatible with Java EE 8 specifications and include the same APIs and Javadoc using the same programming model developers have been using for years. The Jakarta EE 8 TCKs are based on and fully compatible with Java EE 8 TCKs. That means enterprise customers will be able to migrate to Jakarta EE 8 without any changes to Java EE 8 applications.
It's a very big deal since it's the first release from the adoption of the Java EE project by the Eclipse Foundation. I'm looking forward to seeing the future of Jakarta EE and how the enterprise Java landscape will evolve.
Jakarta EE is already compatible with some of the major platforms like RedHat Wildfly, Eclipse Glassfish and Open Liberty. On the project website, you can find more information on compatible products.
I have decided to give Jakarta EE a try, using Wildfly, Gradle and Docker. Let's build a sample application.
Build Configuration with Gradle
First of all, the build.gradle file:
plugins {
id 'java'
id 'war'
}
group 'com.thomasvitale'
version '1.0-SNAPSHOT'
sourceCompatibility = 1.8
repositories {
mavenCentral()
}
dependencies {
providedCompile 'jakarta.platform:jakarta.jakartaee-api:8.0.0'
}
war {
archiveName 'jakarta-ee-getting-started.war'
}
REST API with JAX-RS
As an example, let's set up a REST API using JAX-RS. As you can see, it looks exactly like it was done in Java EE.
@ApplicationPath("api")
public class JakartaApplication extends Application {
}
@Path("books")
@ApplicationScoped
public class BookController {
@Inject
private BookService bookService;
@GET
@Produces(MediaType.APPLICATION_JSON)
public Response getAllBooks() {
return Response.ok(bookService.findAllBooks()).build();
}
@GET
@Path("{id}")
@Produces(MediaType.APPLICATION_JSON)
public Response getBookById(@PathParam("id") String id) {
Book book = bookService.findBookById(id);
if (book == null) {
return Response.status(Response.Status.NOT_FOUND).build();
}
return Response.ok(book).build();
}
}
@ApplicationScoped
public class BookService {
private static Map<String, Book> bookRepository = new ConcurrentHashMap<>();
static {
bookRepository.put("1", new Book("1", "Harry Potter"));
bookRepository.put("2", new Book("2", "The Lord of The Rings"));
bookRepository.put("3", new Book("3", "The Golden Compass"));
}
public List<Book> findAllBooks() {
return new ArrayList<>(bookRepository.values());
}
public Book findBookById(String id) {
return bookRepository.get(id);
}
}
@XmlRootElement
public class Book {
private String id;
private String title;
public Book(String id, String title) {
this.id = id;
this.title = title;
}
}
Deployment
Now it's time to deploy our sample Jakarta EE application. You can choose your favourite platform and deployment strategy. For this example, let's go with Wildfly 17.0.1.Final and Docker. Here it is the Dockerfile:
FROM jboss/wildfly:17.0.1.Final
COPY build/libs/jakarta-ee-getting-started.war /opt/jboss/wildfly/standalone/deployments/
After the deployment, we can access the REST API. For example, if we bind the container 8080 port to the localhost 8080 port, we can get all the books by visiting the URL:
http://localhost:8080/jakarta-ee-getting-started/api/books
Conclusion
Since Jakarta EE 8 is fully compatible with Java EE, getting started with it is straightforward and painless. You can find on my GitHub the source code for the sample application built in this article.
Have you already tried Jakarta EE? What do you think about its future?
If you're interested in cloud native development with Spring Boot and Kubernetes, check out my book Cloud Native Spring in Action.